Optimizing React.js apps for search engines starts with learning React SEO basics. This guide offers practical steps to boost rankings and visibility. Whether you’re making a blog, e-commerce site, or app, these tips help search engines get your content.
Search engines crawl websites differently than users, especially on JavaScript-heavy sites like React apps. This article explains how to overcome this. You’ll discover ways to make your site faster, more discoverable, and friendly for both bots and visitors.
Key Takeaways
- Server-side rendering fixes React’s default SEO challenges.
- Meta tags directly impact how search results display your pages.
- Performance tweaks like code splitting boost both rankings and user experience.
- Structured data helps search engines interpret your content correctly.
- Regular audits keep your React SEO strategy up-to-date with algorithm changes.
Understanding SEO in React.js
SEO in React.js makes your app easy to find by search engines. It optimizes things like routing and content loading. This way, search bots can crawl and rank your site well. React.js SEO strategies help your app work great for both users and search engines.
What is SEO in React.js?
React.js creates dynamic sites, but search engines find JavaScript-heavy content hard to read. React.js SEO strategies help by pre-rendering pages and making metadata accessible. It’s like translating your app into a language search engines get.
Why It Matters for Your Website
Ignoring SEO can keep your content hidden from search results. Here’s why it’s key:
- Improved visibility boosts organic traffic
- Higher rankings mean more customers find you
- Future-proofing your site against algorithm changes
“SEO isn’t an afterthought—it’s part of the foundation for any React app aiming to succeed online.”
Without these strategies, your site might rank lower, lose visitors, or miss target audiences. By focusing on React.js SEO strategies, you make your app friendly to search engines.
Setting Up Your React Environment
Starting with a strong React development setup is key for SEO-friendly coding. First, install the latest Node.js and npm. Then, use Create React App or Vite to quickly set up your project. These tools offer optimized settings for performance and bundling.
- Install Node.js from nodejs.org.
- Run npx create-react-app my-project to generate a starter template.
- Add SEO-focused plugins like react-helmet for meta tags.
Organize your project with clear component folders and a src directory. Use CSS-in-JS libraries like styled-components to keep styles organized. Enable code splitting in Webpack to speed up initial load times—a crucial SEO factor. Use Babel presets targeting modern browsers for compatibility.
“Clean code structures make SEO optimization 50% easier.” – Frontend Weekly Report
For advanced setups, tweak Webpack manually for better caching and asset management. Add ESLint and Prettier early to keep coding standards consistent. Testing frameworks like Jest help find SEO-breaking bugs early in the React development setup.
Getting your environment ready this way makes it easier to add future SEO strategies. Test your setup with npm run build to check if it’s production-ready before moving to server-side rendering steps.
Implementing Server-Side Rendering in React
Learning React server-side rendering makes your site load faster and rank better in search engines. It sends fully ready HTML to users. This helps both search bots and visitors enjoy your site more.
Understanding SSR
Server-side rendering (SSR) creates HTML on the server before sending it to browsers. This is different from client-side rendering. It makes your site load faster and helps search engines find dynamic content.
For React developers, using SSR means better search rankings and user experiences.
Best Libraries for SSR
Library | Key Features | SEO Benefits |
---|---|---|
Next.js | Built-in SSR, static exports | Automatic HTML generation |
Gatsby | Static site generation | Pre-rendered pages for crawlers |
Remix | Route-based rendering | Server-first architecture |
Getting Started with Next.js
Next.js makes React server-side rendering easy with these steps:
- Install via `npx create-next-app`
- Create pages in `/pages` for automatic routing
- Add `getServerSideProps` for dynamic data fetching
Deploy to platforms like Vercel for easy setup. Testing in dev mode shows the rendered HTML in the browser’s source code.
Client-Side Considerations for SEO
Improving client-side SEO is key to making your React app visible to search engines. Server-side rendering helps with the initial load. But, dynamic content and JavaScript need extra care.
First, make sure all important content loads fast. Search engines love quick sites. Cut down on big scripts and focus on content above the fold. Use Google Lighthouse to check how fast your site loads and find slow spots.
- Lazy-load non-critical components to avoid blocking initial renders.
- Use React Router with proper URL structures to maintain clean, crawlable paths.
- Test dynamic content with tools like Fetch API to ensure it’s accessible to crawlers.
Challenge | Solution |
---|---|
JavaScript dependency | Pre-render content or use dynamic rendering services. |
Internal linking | Implement programmatic links with React Router for crawlers to follow. |
Good client-side SEO means avoiding too many redirects and making sure each page has its own meta tags. React’s dynamic nature is great, but don’t forget about SEO. Make sure your site is easy for users and search engines to navigate to keep your rankings high.
Optimizing Meta Tags in React Applications
Meta tags are crucial for your React app’s SEO. They help search engines and social platforms understand your content. By optimizing these tags, your pages will stand out to both users and algorithms. Let’s explore how to make these tags work better for you.
Crafting Effective Meta Descriptions
Meta descriptions are your first chance to grab visitors’ attention. Here’s how to make them effective:
- Keep descriptions under 160 characters to avoid truncation
- Use action verbs like “Explore” or “Discover” to prompt clicks
- Include primary keywords naturally, like “React SEO tools”
Dynamic Meta Tags
Dynamic React apps need more than static meta tags. Tools like react-helmet or next/head help update tags for each page. For example:
import { Helmet } from ‘react-helmet’;
This code snippet allows dynamic title and description changes as users navigate.
Improving Social Sharing
Social platforms use optimized meta tags React for previews. Add Open Graph tags like:
Meta property=”og:title” content=”Your Page Title”
Twitter cards and Pinterest metadata also boost engagement. Test previews with Facebook’s Sharing Debugger to ensure accuracy.
Enhancing Performance for Better Rankings
Improved React performance boosts your site’s ranking on search engines. Faster sites keep users engaged and earn better SEO scores. Here are three ways to make your React app run smoother.
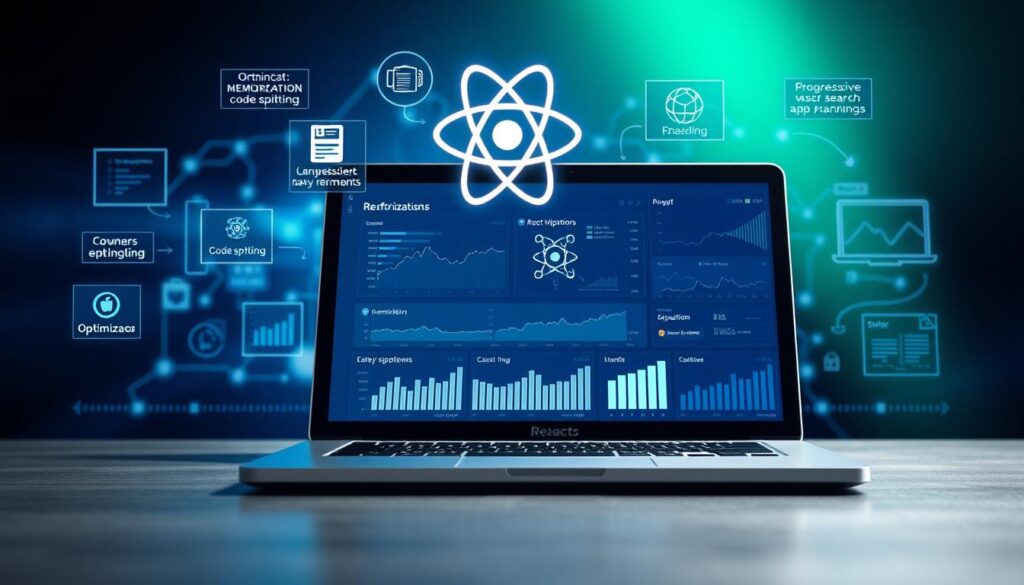
Code Splitting Techniques
Split big bundles into smaller parts to load only what’s needed. Use React.lazy() for dynamic imports or tools like Webpack. This makes your site load faster without losing features.
- Dynamic imports with React.lazy()
- Split by routes or features
- Minimize unused code with tree shaking
Lazy Loading Images
Don’t let images slow down your site. Use the loading=”lazy” attribute or react-lazyload for lazy loading. Focus on content above the fold while loading offscreen images as users scroll.
Browser Caching Strategies
Make repeat visits faster with caching. Set cache headers for static assets and use service workers for dynamic data. Next.js makes cache control easy, while Nginx/XHR handle backend caching well.
These strategies together improve your site’s core web vitals. Focus on these steps to keep your site fast and attractive to search engines.
Utilizing React Plugins for SEO
Boost your React site’s SEO with the best SEO plugins for React. These tools make tasks like dynamic meta tags, sitemaps, and crawlability easier. Let’s look at the top picks to make your work smoother.
- React Helmet Async: Easily manage meta tags, making sure search engines get the latest content.
- Next.js Built-in SEO Features: Use Next.js plugins to automatically create sitemaps and optimize static site generation.
- React Sitemap Generator: Create XML sitemaps automatically for better crawlability.
- React-remark: Improve markdown content for SEO with built-in checks and keywords.
These plugins save time and cut down on mistakes. For instance, SEO plugins for React can update meta tags automatically or create structured data markup. Focus on tools that fit well with your workflow—no need for complicated setups.
Choose plugins that match your project’s needs. Begin with small steps, test changes, and watch how they perform. These tools are most effective when combined with great content and a good user experience. Let the plugins handle the technical stuff while you create valuable content.
Content Strategy and React Integration
Effective component-based content strategy is key to aligning React’s modular design with your SEO goals. By breaking content into reusable components, you streamline updates. This ensures every page element supports search engines and readers.
Start by mapping content needs to React components—think headers, blogs, or product cards. This boosts consistency and scalability.
Begin with modular design principles. For example, create a “HeroSection” component for landing pages or “BlogPost” for articles. This structure lets you tweak SEO metadata or layouts in one place.
Tools like Storybook can preview components in isolation. This ensures they rank well individually.
Leveraging Component-Based Content
- Organize content into reusable modules for faster updates and uniform SEO tags.
- Use dynamic imports to load components only when needed, improving load times.
Maintaining Readability
Ensure components prioritize user experience. Keep text concise and use semantic HTML tags like <article> or <header> to guide search engines. Test readability with tools like Yoast or Lighthouse to avoid dense blocks of text.
User Engagement Tips
“Modular content isn’t just code—it’s a way to engage users with consistent, purpose-built experiences.”
Incorporate interactive elements like collapsible FAQs or scroll-triggered animations. Pair components with clear CTAs to turn visitors into engaged readers. For instance, a “CallToAction” component can standardize buttons across pages while adapting text for different sections.
SEO Testing and Analytics Tools
Regular checks with the right SEO testing tools keep your React site optimized. These tools track rankings, find issues, and measure user behavior. Let’s look at top solutions to help you monitor performance.
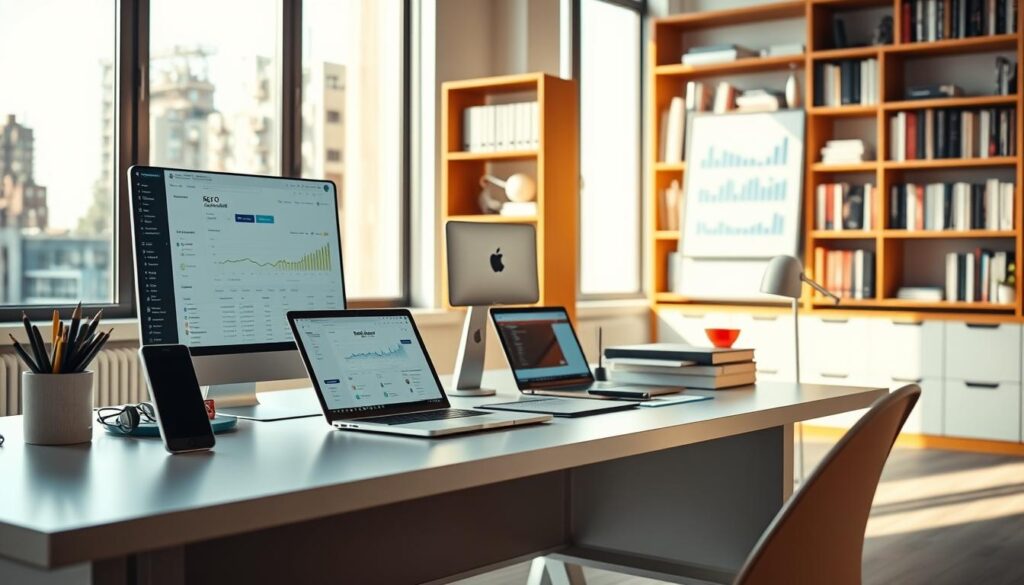
Tools like Google Analytics and Screaming Frog provide critical data. Use them to spot broken links, slow pages, and missing meta tags. Here’s how they help:
- Google Search Console: Identifies crawl errors and mobile usability issues.
- Lighthouse: Audits performance and SEO in Chrome DevTools.
- Ahrefs: Analyzes backlinks and keyword rankings.
A table comparing key features:
Tool | Key Features | Use Case |
---|---|---|
Google Analytics | Session tracking, traffic sources | Measure organic traffic and user engagement |
Screaming Frog | Crawls websites for SEO issues | Identify technical SEO problems |
Lighthouse | Performance audits | Optimize page speed and accessibility |
Testing isn’t a one-time task. Use these tools weekly to spot trends and adjust strategies. For example, track how new meta tags or image optimizations impact rankings. Regular checks ensure your React app stays competitive.
“Data-driven decisions are the backbone of SEO success.”
Pair tools like GTmetrix for speed tests with analytics dashboards. Adjust your site based on real data to boost rankings and user experience.
Advanced Optimization Techniques for React
Take your React site to the next level with Advanced React optimization strategies. These methods keep your site competitive in search rankings and ensure smooth user experiences. Let’s explore three key areas to focus on:
Progressive Web Apps (PWAs)
Make your React app a PWA to enhance speed and engagement. PWAs are quick on mobile, work offline, and show up as app-style icons. Next.js makes setting up PWAs easy, adding service workers and caching for fast loads. This boosts user retention and SEO scores.
Structured Data Implementation
Use schema markup to help search engines grasp your content. For instance, product pages get rich snippets with prices and reviews. Mark up articles, events, or recipes in React components using the JSON-LD format. Google’s Schema.org has tags for any content type.
Monitoring and Debugging
Use tools like Lighthouse and Google Search Console to track performance. They spot issues like slow loading or broken links. Fix these first, then work on layout shifts and interactive metrics. Regular checks keep your site up-to-date with SEO changes.
Mastering these techniques will protect your React site from changing search standards. Every improvement matters—start small and grow as you become more confident!
Ongoing Maintenance and Updates
Optimizing your React.js site is an ongoing task. Continuous SEO updates are crucial to stay ahead. Small changes can greatly improve rankings and user experience.
Regular Performance Audits
Do audits every quarter to check:
- Page speed metrics
- Broken links
- Crawl errors in Google Search Console
Content Refresh Strategies
Outdated content loses relevance. Try these steps:
- Update old blog posts with fresh data
- Add new pages for trending topics
- Remove low-value pages
Tracking SEO Trends
Use these tools to stay informed:
Tool | Purpose |
---|---|
Google Trends | Identify rising keywords |
Ahrefs | Track competitor rankings |
SEMrush | Monitor backlink health |
“The web evolves daily. Adapt or fall behind.” — SEO Experts
Making continuous SEO updates a routine keeps your site visible. Focus on audits, fresh content, and learning new trends to stay competitive.
Conclusion
Mastering React.js SEO means using strategies like server-side rendering and dynamic meta tags. You should also stay active in your efforts. Next, track how these changes affect your rankings with regular SEO result analysis.
Use tools like Google Search Console to find indexing problems or changes in traffic. Every time you update your components, check your performance metrics. This includes things like image lazy loading and structured data.
SEO is a continuous process. Set up monthly audits with tools like Lighthouse to keep your site fast and easy to use. As trends change, update your content strategy to meet user needs.
Whether you’re improving existing pages or adding new features, test how changes affect your visibility. Even small updates, like better meta descriptions or faster component loading, can help more people find you. By combining technical React skills with ongoing SEO result analysis, your site will stay ahead and user-friendly.
FAQ
What is SEO and why is it important for React.js applications?
SEO, or Search Engine Optimization, makes your website more visible in search results. For React.js apps, it’s key because it helps search engines find your dynamic content. This makes it easier for people to find your site.
How can I optimize my React.js app for search engines?
To boost your React.js app’s SEO, use Server-Side Rendering (SSR). Also, optimize your meta tags and use React plugins. Improve site speed with lazy loading and code splitting.
What is Server-Side Rendering, and how does it help with SEO?
Server-Side Rendering (SSR) means React components are rendered on the server. This makes it easier for search engines to crawl your pages. It also makes your app load faster for users.
How do meta tags affect SEO in React applications?
Meta tags give search engines important info about your web pages. Writing good meta descriptions and updating them helps both search engines and users. This boosts click-through rates.
What are some recommended React plugins for SEO?
For SEO, use React Helmet to manage your document head. Also, React Router helps create SEO-friendly navigation. These tools make SEO easier in your projects.
How can performance optimization improve my website’s search ranking?
Improving your site’s performance with code splitting and lazy loading helps. It also makes your site faster. Faster sites rank better in search results.
What strategies can I use for ongoing SEO maintenance?
For ongoing SEO, do regular performance audits and update old content. Stay current with SEO trends. This keeps your site competitive and appealing to users and search engines.
Leave a Reply